Select a language
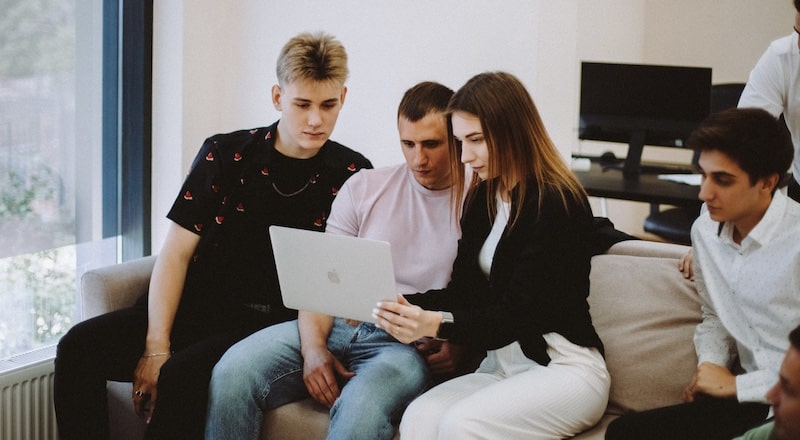
Websocket API on NodeJS
Websocket API on NodeJS
In this article, we will look at the Websocket technology for developing applications on nodejs.
First of all, we need to understand what a Websocket is and what it is for.
Websocket is one of the communication protocols used on top of a TCP connection, which is necessary for the exchange of messages between the browser and the web server via a persistent connection. Data, in turn, is transmitted in both directions in the form of "packets", without breaking the connection, without additional HTTP requests.
This technology is necessary for services that require constant data exchange. These can be both games and real-time chats. This technology is used in many web applications and the ability to develop using this protocol can be a very useful skill for a developer.
With its help, you can implement a large amount of functionality, instant notification transmission for the user and much more.
One of the main advantages is that this technology is quite easy to use, having learned how to handle it once, then working with it will not cause any difficulties.
It is also not necessary to build the entire server application using this technology, you can implement it gradually, only if necessary in specific places of functionality.
How to implement and apply Websocket technology in Nodejs?
Nodejs has a number of libraries that make it possible to build interaction between the client and the server using the Websocket protocol on top of the native HTTP implementation. ws or an even more popular library can be singled out as examples of such libraries socket.io . Let's analyze the implementation of Websocket in nodejs using the example of the ws library.
The first step is to install this library in the project, for this there are several commands: yarn add ws or npm i ws.
Use the necessary command depending on your package manager.
Next, after installation, you need to connect this library using the following command:
const webSocket = require('ws');
After that, we will create an instance of the WebSocket class, specify the port on which our WebSocket server will be launched:
const server = new webSocket.Server({port: 8000});
The approaches of the HTTP server and WebSocket server differ greatly in the following: the HTTP server accepts all requests directly, and the server using WebSocket technology accepts requests from the connection, while the connection itself is full-duplex.
What is a full duplex state?
Duplex and half—duplex are the modes of operation of receiving and transmitting devices.
If we are talking about duplex mode, devices can transmit and receive information simultaneously.
And in the half—duplex mode - either transmit or receive information.
Next, we need to write a connection handler using the OnConnection function:
server.on(‘connection', onConnection);
Now we are implementing the connection of two event handlers for wsClient objects: message and close.
message - this parameter handles the event of an incoming message from the client.
close is an event for disconnecting the connection with the client.
In our OnConnection function, we will write any message in the console and send it to the client:
function onConnect(wsClient) {
console.log(‘New message‘);
wsClient.send(‘Hello world‘);
wsClient.on('message', function(message) {
/* тут описывается обработка сообщений от клиента */
}
wsClient.on('close', function() {
console.log(‘Closed‘);
}
}
This is how we implemented sending messages on the server side.
If the close event occurs, we will be notified about it in the console.
But it is not enough for us to describe all the logic only on the server side, we also need to configure this library and receive data on the client.
We add the library using the package manager used on the client, similar to the example on the server, and then proceed to the connection process.
Enabling technology on the client side:
const clientSocket = new WebSocket('ws://localhost:8000');
clientSocket.onopen = function () {
console.log(‘Server was connected’);
};
Next, we need to describe the command to receive data from the server on the client so that we can exchange data in real time:
try {
const newMessage = JSON.parse(message);
wsClient.send(newMessage.data);
} catch (error) {
console.log('Ошибка', error);
}
Thus, we have configured WebSocket on the client and on the server.
Now we can continue to supplement our functionality with various additional functions. We can describe additional middleware before using our logic on WebSocket, and we can also create various messages or organize sending notifications and receiving all this on the client side.
In this article, we got acquainted with the WebSocket API technology on nodejs and learned how to implement it into projects.